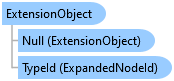
'Declaration
<DataContractAttribute("http://opcfoundation.org/UA/2008/02/Types.xsd")> Public Class ExtensionObject
'Usage
Dim instance As ExtensionObject
[DataContract("http://opcfoundation.org/UA/2008/02/Types.xsd")] public class ExtensionObject
[DataContract("http://opcfoundation.org/UA/2008/02/Types.xsd")] public ref class ExtensionObject
This class is a wrapper/helper class for storing data types that a receiver might not understand, or be prepared to handle. This class may use System.Reflection to analyze an object and retrieve the values of its public properties directly, and then encode those into a string representation that can then be easily encoded as a single string.
An instance of the ExtensionObject is a container for any complex data types which cannot be encoded as one of the other built-in data types. The ExtensionObject contains a complex value serialized as a sequence of bytes or as an XML element. It also contains an identifier which indicates what data it contains and how it is encoded.
The following example demonstrates a simple class containing 3 public properties of type int, DateTime and string. This class implements the IEncodeable interface, and is then encoded using the WriteExtensionObject method.
//First, we will define a very simple class object that will represent //some real-world process. class simpleClass : IEncodeable { //fields public string PublicFieldNotVisible = "I should not be encoded"; //properties private string stringField; public string StringProperty { get { return (stringField); } set { stringField = value; } } private int intField; public int IntProperty { get { return (intField); } set { intField = value; } } private DateTime datetimeField; public DateTime DatetimeProperty { get { return (datetimeField); } set { datetimeField = value; } } //class constructor public simpleClass(string StringValue, int IntValue, DateTime DateTimeValue) { StringProperty = StringValue; IntProperty = IntValue; DatetimeProperty = DateTimeValue; } public simpleClass(simpleClass SimpleClassInstance) { StringProperty = SimpleClassInstance.StringProperty; IntProperty = SimpleClassInstance.IntProperty; DatetimeProperty = SimpleClassInstance.DatetimeProperty; } #region IEncodeable Members public ExpandedNodeId TypeId { get { return (new ExpandedNodeId(Guid.NewGuid())); } } public void Encode(IEncoder encoder) { if (encoder != null) { //our simple object has 3 properies: string, int and datetime encoder.WriteString("StringProperty", this.StringProperty); encoder.WriteInt32("IntProperty", this.IntProperty); encoder.WriteDateTime("DateTimeProperty", this.DatetimeProperty); } } public void Decode(IDecoder decoder) { if (decoder != null) { this.StringProperty = decoder.ReadString("StringProperty"); this.IntProperty = decoder.ReadInt16("IntProperty"); this.DatetimeProperty = decoder.ReadDateTime("DateTimeProperty"); } } public bool IsEqual(IEncodeable encodeable) { return (encodeable.Equals(this)); } #endregion #region ICloneable Members public new object MemberwiseClone() { return (new simpleClass(this)); } #endregion } public void EncodeExample() { //define an instance of our class object, defined above. simpleClass mySimpleClassInstance1 = new simpleClass("String", int.MaxValue, DateTime.Now); //define an object that will encapsulate/extend our simple instance above ExtensionObject extendedSimpleClassInstance = new ExtensionObject(mySimpleClassInstance1); /// //encode our class object into the stream uaEncoderInstance.WriteExtensionObject( "Extended1", extendedSimpleClassInstance); }
'First, we will define a very simple class object that will represent 'some real-world process. Class simpleClass Inherits IEncodeable 'fields Public PublicFieldNotVisible As String = "I should not be encoded" 'properties Private stringField As String Private intField As Integer Private datetimeField As DateTime 'class constructor Public Sub New(ByVal StringValue As String, ByVal IntValue As Integer, ByVal DateTimeValue As DateTime) StringProperty = StringValue IntProperty = IntValue DatetimeProperty = DateTimeValue End Sub Public Sub New(ByVal SimpleClassInstance As simpleClass) StringProperty = SimpleClassInstance.StringProperty IntProperty = SimpleClassInstance.IntProperty DatetimeProperty = SimpleClassInstance.DatetimeProperty End Sub Public Property StringProperty As String Get Return stringField End Get Set stringField = value End Set End Property Public Property IntProperty As Integer Get Return intField End Get Set intField = value End Set End Property Public Property DatetimeProperty As DateTime Get Return datetimeField End Get Set datetimeField = value End Set End Property Public ReadOnly Property TypeId As ExpandedNodeId Get Return New ExpandedNodeId(Guid.NewGuid) End Get End Property Public Sub Encode(ByVal encoder As IEncoder) If encoder Isnot Nothing Then 'our simple object has 3 properies: string, int and datetime encoder.WriteString("StringProperty", Me.StringProperty) encoder.WriteInt32("IntProperty", Me.IntProperty) encoder.WriteDateTime("DateTimeProperty", Me.DatetimeProperty) End If End Sub Public Sub Decode(ByVal decoder As IDecoder) If decoder Isnot Nothing Then Me.StringProperty = decoder.ReadString("StringProperty") Me.IntProperty = decoder.ReadInt16("IntProperty") Me.DatetimeProperty = decoder.ReadDateTime("DateTimeProperty") End If End Sub Public Function IsEqual(ByVal encodeable As IEncodeable) As Boolean Return encodeable.Equals(Me) End Function Public Function Clone() As Object Return New simpleClass(Me) End Function End Class Public Sub nodeid() 'define an instance of our class object, defined above. Dim mySimpleClassInstance1 As simpleClass = New simpleClass("String", int.MaxValue, DateTime.Now) 'define an object that will encapsulate/extend our simple instance above Dim extendedSimpleClassInstance As ExtensionObject = New ExtensionObject(mySimpleClassInstance1) 'encode our class object into the stream uaEncoderInstance.WriteExtensionObject("Extended1", extendedSimpleClassInstance) End Sub
System.Object
Opc.Ua.ExtensionObject
Target Platforms: Windows 7, Windows Vista SP1 or later, Windows XP SP3, Windows Server 2008 (Server Core not supported), Windows Server 2008 R2 (Server Core supported with SP1 or later), Windows Server 2003 SP2