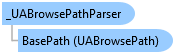
'Declaration
<CLSCompliantAttribute(False)> <ComVisibleAttribute(True)> <GuidAttribute("7535B40D-25FC-4689-93FC-DA9D3C0654FB")> <InterfaceTypeAttribute(ComInterfaceType.InterfaceIsDual)> Public Interface _UABrowsePathParser
'Usage
Dim instance As _UABrowsePathParser
[CLSCompliant(false)] [ComVisible(true)] [Guid("7535B40D-25FC-4689-93FC-DA9D3C0654FB")] [InterfaceType(ComInterfaceType.InterfaceIsDual)] public interface _UABrowsePathParser
[CLSCompliant(false)] [ComVisible(true)] [Guid("7535B40D-25FC-4689-93FC-DA9D3C0654FB")] [InterfaceType(ComInterfaceType.InterfaceIsDual)] public interface class _UABrowsePathParser
This member or type is for use from COM. It is not meant to be used from .NET or Python. Refer to the corresponding .NET member or type instead, if you are developing in .NET or Python.
// Parses an absolute OPC-UA browse path and displays its starting node and elements. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class procedure Parse.Main; var BrowsePath: _UABrowsePath; BrowsePathElement: _UABrowsePathElement; BrowsePathParser: OpcLabs_EasyOpcUA_TLB._UABrowsePathParser; Count: Cardinal; Element: OleVariant; ElementEnumerator: IEnumVariant; begin BrowsePathParser := CoUABrowsePathParser.Create; try BrowsePath := BrowsePathParser.Parse('[ObjectsFolder]/Data/Static/UserScalar'); except on E: EOleException do begin WriteLn(Format('*** Failure: %s', [E.GetBaseException.Message])); Exit; end; end; // Display results WriteLn('StartingNodeId: ', BrowsePath.StartingNodeId.ToString); WriteLn('Elements:'); ElementEnumerator := BrowsePath.Elements.GetEnumerator; while (ElementEnumerator.Next(1, Element, Count) = S_OK) do begin BrowsePathElement := IUnknown(Element) as _UABrowsePathElement; WriteLn(BrowsePathElement.ToString); end; // Example output: // StartingNodeId: ObjectsFolder // Elements: // /Data // /Static // /UserScalar end;
// Parses an absolute OPC-UA browse path and displays its starting node and elements. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. $BrowsePathParser = new COM("OpcLabs.EasyOpc.UA.Navigation.Parsing.UABrowsePathParser"); try { $BrowsePath = $BrowsePathParser->Parse("[ObjectsFolder]/Data/Static/UserScalar"); } catch (com_exception $e) { printf("*** Failure: %s\n", $e->getMessage()); exit(); } // Display results printf("StartingNodeId: %s\n", $BrowsePath->StartingNodeId); printf("Elements:\n"); for ($i = 0; $i < $BrowsePath->Elements->Count; $i++) { printf("%s\n", $BrowsePath->Elements[$i]); } // Example output: // StartingNodeId: ObjectsFolder // Elements: // /Data // /Static // /UserScalar
REM Parses an absolute OPC-UA browse path and displays its starting node and elements. REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. Private Sub Parse_Main_Command_Click() OutputText = "" Dim BrowsePathParser As New UABrowsePathParser On Error Resume Next Dim browsePath As UABrowsePath Set browsePath = BrowsePathParser.Parse("[ObjectsFolder]/Data/Static/UserScalar") If Err.Number <> 0 Then OutputText = OutputText & "*** Failure: " & Err.Source & ": " & Err.Description & vbCrLf Exit Sub End If On Error GoTo 0 ' Display results OutputText = OutputText & "StartingNodeId: " & browsePath.StartingNodeId & vbCrLf OutputText = OutputText & "Elements:" & vbCrLf Dim BrowsePathElement: For Each BrowsePathElement In browsePath.Elements OutputText = OutputText & BrowsePathElement & vbCrLf Next ' Example output: 'StartingNodeId: ObjectsFolder 'Elements: '/Data '/Static '/UserScalar End Sub
Rem Parses an absolute OPC-UA browse path and displays its starting node and elements. Rem Rem Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . Rem OPC client and subscriber examples in VBScript on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBScript . Rem Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own Rem a commercial license in order to use Online Forums, and we reply to every post. Option Explicit Dim BrowsePathParser: Set BrowsePathParser = CreateObject("OpcLabs.EasyOpc.UA.Navigation.Parsing.UABrowsePathParser") On Error Resume Next Dim BrowsePath: Set BrowsePath = BrowsePathParser.Parse("[ObjectsFolder]/Data/Static/UserScalar") If Err.Number <> 0 Then WScript.Echo "*** Failure: " & Err.Source & ": " & Err.Description WScript.Quit End If On Error Goto 0 ' Display results WScript.Echo "StartingNodeId: " & BrowsePath.StartingNodeId WScript.Echo "Elements:" Dim BrowsePathElement: For Each BrowsePathElement In BrowsePath.Elements WScript.Echo BrowsePathElement Next
// Parses a relative OPC-UA browse path and displays its elements. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class procedure ParseRelative.Main; var BrowsePathElement: _UABrowsePathElement; BrowsePathElements: _UABrowsePathElementCollection; BrowsePathParser: OpcLabs_EasyOpcUA_TLB._UABrowsePathParser; Count: Cardinal; Element: OleVariant; ElementEnumerator: IEnumVariant; begin BrowsePathParser := CoUABrowsePathParser.Create; try BrowsePathElements := BrowsePathParser.ParseRelative('/Data.Dynamic.Scalar.CycleComplete'); except on E: EOleException do begin WriteLn(Format('*** Failure: %s', [E.GetBaseException.Message])); Exit; end; end; // Display results ElementEnumerator := BrowsePathElements.GetEnumerator; while (ElementEnumerator.Next(1, Element, Count) = S_OK) do begin BrowsePathElement := IUnknown(Element) as _UABrowsePathElement; WriteLn(BrowsePathElement.ToString); end; // Example output: // /Data // .Dynamic // .Scalar // .CycleComplete end;
// Parses a relative OPC-UA browse path and displays its elements. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. $BrowsePathParser = new COM("OpcLabs.EasyOpc.UA.Navigation.Parsing.UABrowsePathParser"); try { $BrowsePathElements = $BrowsePathParser->ParseRelative("/Data.Dynamic.Scalar.CycleComplete"); } catch (com_exception $e) { printf("*** Failure: %s\n", $e->getMessage()); exit(); } // Display results for ($i = 0; $i < $BrowsePathElements->Count; $i++) { printf("%s\n", $BrowsePathElements[$i]); } // Example output: // /Data // .Dynamic // .Scalar // .CycleComplete
REM Parses a relative OPC-UA browse path and displays its elements. REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. Private Sub ParseRelative_Main_Command_Click() OutputText = "" Dim BrowsePathParser As New UABrowsePathParser On Error Resume Next Dim BrowsePathElements As UABrowsePathElementCollection Set BrowsePathElements = BrowsePathParser.ParseRelative("/Data.Dynamic.Scalar.CycleComplete") If Err.Number <> 0 Then OutputText = OutputText & "*** Failure: " & Err.Source & ": " & Err.Description & vbCrLf Exit Sub End If On Error GoTo 0 ' Display results Dim BrowsePathElement: For Each BrowsePathElement In BrowsePathElements OutputText = OutputText & BrowsePathElement & vbCrLf Next ' Example output: '/Data '.Dynamic '.Scalar '.CycleComplete End Sub
Rem Parses a relative OPC-UA browse path and displays its elements. Rem Rem Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . Rem OPC client and subscriber examples in VBScript on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBScript . Rem Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own Rem a commercial license in order to use Online Forums, and we reply to every post. Option Explicit Dim BrowsePathParser: Set BrowsePathParser = CreateObject("OpcLabs.EasyOpc.UA.Navigation.Parsing.UABrowsePathParser") On Error Resume Next Dim BrowsePathElements: Set BrowsePathElements = BrowsePathParser.ParseRelative("/Data.Dynamic.Scalar.CycleComplete") If Err.Number <> 0 Then WScript.Echo "*** Failure: " & Err.Source & ": " & Err.Description WScript.Quit End If On Error Goto 0 ' Display results Dim BrowsePathElement: For Each BrowsePathElement In BrowsePathElements WScript.Echo BrowsePathElement Next
// Attempts to parse an absolute OPC-UA browse path and displays its starting node and elements. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class procedure TryParse.Main; var BrowsePath: _UABrowsePath; BrowsePathElement: _UABrowsePathElement; BrowsePathParser: OpcLabs_EasyOpcUA_TLB._UABrowsePathParser; BrowsePathResult: OleVariant; Count: Cardinal; Element: OleVariant; ElementEnumerator: IEnumVariant; StringParsingError: _StringParsingError; begin BrowsePathParser := CoUABrowsePathParser.Create; StringParsingError := BrowsePathParser.TryParse('[ObjectsFolder]/Data/Static/UserScalar', BrowsePathResult); // Display results if StringParsingError <> nil then begin WriteLn('*** Error: ', StringParsingError.ToString); Exit; end; BrowsePath := IUnknown(BrowsePathResult) as _UABrowsePath; WriteLn('StartingNodeId: ', BrowsePath.StartingNodeId.ToString); WriteLn('Elements:'); ElementEnumerator := BrowsePath.Elements.GetEnumerator; while (ElementEnumerator.Next(1, Element, Count) = S_OK) do begin BrowsePathElement := IUnknown(Element) as _UABrowsePathElement; WriteLn(BrowsePathElement.ToString); end; // Example output: // StartingNodeId: ObjectsFolder // Elements: // /Data // /Static // /UserScalar end;
// Attempts to parses an absolute OPC-UA browse path and displays its starting node and elements. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. $BrowsePath = new COM("OpcLabs.EasyOpc.UA.Navigation.UABrowsePath"); $BrowsePathParser = new COM("OpcLabs.EasyOpc.UA.Navigation.Parsing.UABrowsePathParser"); $StringParsingError = $BrowsePathParser->TryParse("[ObjectsFolder]/Data/Static/UserScalar", $BrowsePath); // Display results if (!is_null($StringParsingError)) { printf("*** Error: %s\n", $StringParsingError); exit(); } printf("StartingNodeId: %s\n", $BrowsePath->StartingNodeId); printf("Elements:\n"); for ($i = 0; $i < $BrowsePath->Elements->Count; $i++) { printf("%s\n", $BrowsePath->Elements[$i]); } // Example output: // StartingNodeId: ObjectsFolder // Elements: // /Data // /Static // /UserScalar
REM Attempts to parses an absolute OPC-UA browse path and displays its starting node and elements. REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. Private Sub TryParse_Main_Command_Click() OutputText = "" Dim BrowsePathParser As New UABrowsePathParser Dim stringParsingError As stringParsingError Dim browsePath As Variant Set stringParsingError = BrowsePathParser.TryParse("[ObjectsFolder]/Data/Static/UserScalar", browsePath) ' Display results If Not stringParsingError Is Nothing Then OutputText = OutputText & "*** Error: " & stringParsingError & vbCrLf Exit Sub End If OutputText = OutputText & "StartingNodeId: " & browsePath.StartingNodeId & vbCrLf OutputText = OutputText & "Elements:" & vbCrLf Dim BrowsePathElement: For Each BrowsePathElement In browsePath.Elements OutputText = OutputText & BrowsePathElement & vbCrLf Next ' Example output: 'StartingNodeId: ObjectsFolder 'Elements: '/Data '/Static '/UserScalar End Sub
Rem Attempts to parses an absolute OPC-UA browse path and displays its starting node and elements. Rem Rem Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . Rem OPC client and subscriber examples in VBScript on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBScript . Rem Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own Rem a commercial license in order to use Online Forums, and we reply to every post. Option Explicit Dim BrowsePathParser: Set BrowsePathParser = CreateObject("OpcLabs.EasyOpc.UA.Navigation.Parsing.UABrowsePathParser") Dim BrowsePath Dim StringParsingError: Set StringParsingError = BrowsePathParser.TryParse("[ObjectsFolder]/Data/Static/UserScalar", BrowsePath) ' Display results If Not (StringParsingError Is Nothing) Then WScript.Echo "*** Error: " & StringParsingError WScript.Quit End If WScript.Echo "StartingNodeId: " & BrowsePath.StartingNodeId WScript.Echo "Elements:" Dim BrowsePathElement: For Each BrowsePathElement In BrowsePath.Elements WScript.Echo BrowsePathElement Next
// Attempts to parse a relative OPC-UA browse path and displays its elements. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class procedure TryParseRelative.Main; var BrowsePathElement: _UABrowsePathElement; BrowsePathElements: _UABrowsePathElementCollection; BrowsePathParser: OpcLabs_EasyOpcUA_TLB._UABrowsePathParser; Count: Cardinal; Element: OleVariant; ElementEnumerator: IEnumVariant; StringParsingError: _StringParsingError; begin BrowsePathElements := CoUABrowsePathElementCollection.Create; BrowsePathParser := CoUABrowsePathParser.Create; StringParsingError := BrowsePathParser.TryParseRelative('/Data.Dynamic.Scalar.CycleComplete', BrowsePathElements); // Display results if StringParsingError <> nil then begin WriteLn('*** Error: ', StringParsingError.ToString); Exit; end; ElementEnumerator := BrowsePathElements.GetEnumerator; while (ElementEnumerator.Next(1, Element, Count) = S_OK) do begin BrowsePathElement := IUnknown(Element) as _UABrowsePathElement; WriteLn(BrowsePathElement.ToString); end; // Example output: // /Data // .Dynamic // .Scalar // .CycleComplete end;
// Attempts to parse a relative OPC-UA browse path and displays its elements. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. $BrowsePathElements = new COM("OpcLabs.EasyOpc.UA.Navigation.UABrowsePathElementCollection"); $BrowsePathParser = new COM("OpcLabs.EasyOpc.UA.Navigation.Parsing.UABrowsePathParser"); $StringParsingError = $BrowsePathParser->TryParseRelative("/Data.Dynamic.Scalar.CycleComplete", $BrowsePathElements); // Display results if (!is_null($StringParsingError)) { printf("*** Error: %s\n", $StringParsingError); exit(); } printf("StartingNodeId: %s\n", $BrowsePath->StartingNodeId); printf("Elements:\n"); for ($i = 0; $i < $BrowsePathElements->Count; $i++) { printf("%s\n", $BrowsePathElements[$i]); } // Example output: // /Data // .Dynamic // .Scalar // .CycleComplete
REM Attempts to parse a relative OPC-UA browse path and displays its elements. REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. Private Sub TryParseRelative_Main_Command_Click() OutputText = "" Dim BrowsePathElements As New UABrowsePathElementCollection Dim BrowsePathParser As New UABrowsePathParser Dim stringParsingError As stringParsingError Set stringParsingError = BrowsePathParser.TryParseRelative("/Data.Dynamic.Scalar.CycleComplete", BrowsePathElements) ' Display results If Not stringParsingError Is Nothing Then OutputText = OutputText & "*** Error: " & stringParsingError & vbCrLf Exit Sub End If Dim BrowsePathElement: For Each BrowsePathElement In BrowsePathElements OutputText = OutputText & BrowsePathElement & vbCrLf Next ' Example output: '/Data '.Dynamic '.Scalar '.CycleComplete End Sub
Rem Attempts to parse a relative OPC-UA browse path and displays its elements. Rem Rem Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . Rem OPC client and subscriber examples in VBScript on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBScript . Rem Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own Rem a commercial license in order to use Online Forums, and we reply to every post. Option Explicit Dim BrowsePathElements: Set BrowsePathElements = CreateObject("OpcLabs.EasyOpc.UA.Navigation.UABrowsePathElementCollection") Dim BrowsePathParser: Set BrowsePathParser = CreateObject("OpcLabs.EasyOpc.UA.Navigation.Parsing.UABrowsePathParser") Dim StringParsingError: Set StringParsingError = BrowsePathParser.TryParseRelative("/Data.Dynamic.Scalar.CycleComplete", BrowsePathElements) ' Display results If Not (StringParsingError Is Nothing) Then WScript.Echo "*** Error: " & StringParsingError WScript.Quit End If Dim BrowsePathElement: For Each BrowsePathElement In BrowsePathElements WScript.Echo BrowsePathElement Next