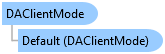
'Declaration
<CLSCompliantAttribute(True)> <ComDefaultInterfaceAttribute(OpcLabs.EasyOpc.DataAccess.Engine.ComTypes._DAClientMode)> <ComVisibleAttribute(True)> <GuidAttribute("093526CF-696F-4986-A4F4-44ED5060AE6B")> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <ValueControlAttribute("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=False, Export=True, PageId=10001)> <SerializableAttribute()> Public Class DAClientMode Inherits OpcLabs.BaseLib.Parameters Implements LINQPad.ICustomMemberProvider, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.ComTypes._Parameters, OpcLabs.EasyOpc.DataAccess.Engine.ComTypes._DAClientMode, System.ComponentModel.INotifyPropertyChanged, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
'Usage
Dim instance As DAClientMode
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.DataAccess.Engine.ComTypes._DAClientMode)] [ComVisible(true)] [Guid("093526CF-696F-4986-A4F4-44ED5060AE6B")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public class DAClientMode : OpcLabs.BaseLib.Parameters, LINQPad.ICustomMemberProvider, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.ComTypes._Parameters, OpcLabs.EasyOpc.DataAccess.Engine.ComTypes._DAClientMode, System.ComponentModel.INotifyPropertyChanged, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.DataAccess.Engine.ComTypes._DAClientMode)] [ComVisible(true)] [Guid("093526CF-696F-4986-A4F4-44ED5060AE6B")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public ref class DAClientMode : public OpcLabs.BaseLib.Parameters, LINQPad.ICustomMemberProvider, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.ComTypes._Parameters, OpcLabs.EasyOpc.DataAccess.Engine.ComTypes._DAClientMode, System.ComponentModel.INotifyPropertyChanged, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
// This example shows how to read an item synchronously, and display its value, timestamp and quality. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using OpcLabs.EasyOpc.DataAccess; using OpcLabs.EasyOpc.OperationModel; namespace DocExamples.DataAccess._EasyDAClient { partial class ReadItem { public static void Synchronous() { // Instantiate the client object. var client = new EasyDAClient(); // Specify that only synchronous method is allowed. By default, both synchronous and asynchronous methods are // allowed, and the component picks a suitable method automatically. Disallowing asynchronous method leaves // only the synchronous method available for selection. client.InstanceParameters.Mode.AllowAsynchronousMethod = false; DAVtq vtq; try { vtq = client.ReadItem("", "OPCLabs.KitServer.2", "Simulation.Random"); } catch (OpcException opcException) { Console.WriteLine("*** Failure: {0}", opcException.GetBaseException().Message); return; } Console.WriteLine("Vtq: {0}", vtq); } } }
' This example shows how to read an item synchronously, and display its value, timestamp and quality. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports OpcLabs.EasyOpc.DataAccess Imports OpcLabs.EasyOpc.OperationModel Namespace DataAccess._EasyDAClient Partial Friend Class ReadItem Public Shared Sub Synchronous() ' Instantiate the client object. Dim client = New EasyDAClient() ' Specify that only synchronous method is allowed. By default, both synchronous and asynchronous methods are ' allowed, and the component picks a suitable method automatically. Disallowing asynchronous method leaves ' only the synchronous method available for selection. client.InstanceParameters.Mode.AllowAsynchronousMethod = False Dim vtq As DAVtq Try vtq = client.ReadItem("", "OPCLabs.KitServer.2", "Simulation.Random") Catch opcException As OpcException Console.WriteLine("*** Failure: {0}", opcException.GetBaseException().Message) Exit Sub End Try Console.WriteLine("Vtq: {0}", vtq) End Sub End Class End Namespace
# This example shows how to read an item synchronously, and display its value, timestamp and quality. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc # Import .NET namespaces. from OpcLabs.EasyOpc import * from OpcLabs.EasyOpc.DataAccess import * from OpcLabs.EasyOpc.OperationModel import * # Instantiate the client object. client = EasyDAClient() # Specify that only synchronous method is allowed. By default, both synchronous and asynchronous methods are # allowed, and the component picks a suitable method automatically. Disallowing asynchronous method leaves # only the synchronous method available for selection. client.InstanceParameters.Mode.AllowAsynchronousMethod = False # Perform the operation. try: vtq = IEasyDAClientExtension.ReadItem(client, '', 'OPCLabs.KitServer.2', 'Simulation.Random') except OpcException as opcException: print('*** Failure: ' + opcException.GetBaseException().Message, sep='') exit() # Display results. print('Vtq: ', vtq, sep='')
// This example shows how to read 4 items at once synchronously, and display their values, timestamps and qualities. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using System.Diagnostics; using OpcLabs.EasyOpc.DataAccess; using OpcLabs.EasyOpc.DataAccess.OperationModel; namespace DocExamples.DataAccess._EasyDAClient { partial class ReadMultipleItems { public static void Synchronous() { // Instantiate the client object. var client = new EasyDAClient(); // Specify that only synchronous method is allowed. By default, both synchronous and asynchronous methods are // allowed, and the component picks a suitable method automatically. Disallowing asynchronous method leaves // only the synchronous method available for selection. client.InstanceParameters.Mode.AllowAsynchronousMethod = false; DAVtqResult[] vtqResults = client.ReadMultipleItems("OPCLabs.KitServer.2", new DAItemDescriptor[] { "Simulation.Random", "Trends.Ramp (1 min)", "Trends.Sine (1 min)", "Simulation.Register_I4" }); for (int i = 0; i < vtqResults.Length; i++) { Debug.Assert(vtqResults[i] != null); if (vtqResults[i].Succeeded) Console.WriteLine("vtqResult[{0}].Vtq: {1}", i, vtqResults[i].Vtq); else Console.WriteLine("vtqResult[{0}] *** Failure: {1}", i, vtqResults[i].ErrorMessageBrief); } } // Example output: // //vtqResult[0].Vtq: 0.00125125888851588 { System.Double} @2020-04-10T15:29:20.642; GoodNonspecific(192) //vtqResult[1].Vtq: 0.344052940607071 {System.Double} @2020-04-10T15:29:20.643; GoodNonspecific(192) //vtqResult[2].Vtq: 0.830410616568378 {System.Double} @2020-04-10T15:29:20.643; GoodNonspecific(192) //vtqResult[3].Vtq: 0 {System.Int32} @1601-01-01T00:00:00.000; GoodNonspecific(192) } }
' This example shows how to read 4 items at once synchronously, and display their values, timestamps and qualities. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports OpcLabs.EasyOpc.DataAccess Imports OpcLabs.EasyOpc.DataAccess.OperationModel Namespace DataAccess._EasyDAClient Partial Friend Class ReadMultipleItems Public Shared Sub Synchronous() ' Instantiate the client object. Dim client = New EasyDAClient() ' Specify that only synchronous method is allowed. By default, both synchronous and asynchronous methods are ' allowed, and the component picks a suitable method automatically. Disallowing asynchronous method leaves ' only the synchronous method available for selection. client.InstanceParameters.Mode.AllowAsynchronousMethod = False Dim vtqResults() As DAVtqResult = client.ReadMultipleItems("OPCLabs.KitServer.2", New DAItemDescriptor() {"Simulation.Random", "Trends.Ramp (1 min)", "Trends.Sine (1 min)", "Simulation.Register_I4"}) For i = 0 To vtqResults.Length - 1 Debug.Assert(vtqResults(i) IsNot Nothing) If vtqResults(i).Succeeded Then Console.WriteLine("vtqResult[{0}].Vtq: {1}", i, vtqResults(i).Vtq) Else Console.WriteLine("vtqResult[{0}] *** Failure: {1}", i, vtqResults(i).ErrorMessageBrief) End If Next i End Sub ' Example output: ' 'vtqResult[0].Vtq: 0.00125125888851588 { System.Double} @2020-04-10T15:29:20.642; GoodNonspecific(192) 'vtqResult[1].Vtq: 0.344052940607071 {System.Double} @2020-04-10T15:29:20.643; GoodNonspecific(192) 'vtqResult[2].Vtq: 0.830410616568378 {System.Double} @2020-04-10T15:29:20.643; GoodNonspecific(192) 'vtqResult[3].Vtq: 0 {System.Int32} @1601-01-01T00:00:00.000; GoodNonspecific(192) End Class End Namespace
Rem This example shows how to read 4 items at once synchronously, and display their values, timestamps and qualities. Rem Rem Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . Rem OPC client and subscriber examples in VBScript on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBScript . Rem Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own Rem a commercial license in order to use Online Forums, and we reply to every post. Option Explicit Dim ReadItemArguments1: Set ReadItemArguments1 = CreateObject("OpcLabs.EasyOpc.DataAccess.OperationModel.DAReadItemArguments") ReadItemArguments1.ServerDescriptor.ServerClass = "OPCLabs.KitServer.2" ReadItemArguments1.ItemDescriptor.ItemID = "Simulation.Random" Dim ReadItemArguments2: Set ReadItemArguments2 = CreateObject("OpcLabs.EasyOpc.DataAccess.OperationModel.DAReadItemArguments") ReadItemArguments2.ServerDescriptor.ServerClass = "OPCLabs.KitServer.2" ReadItemArguments2.ItemDescriptor.ItemID = "Trends.Ramp (1 min)" Dim ReadItemArguments3: Set ReadItemArguments3 = CreateObject("OpcLabs.EasyOpc.DataAccess.OperationModel.DAReadItemArguments") ReadItemArguments3.ServerDescriptor.ServerClass = "OPCLabs.KitServer.2" ReadItemArguments3.ItemDescriptor.ItemID = "Trends.Sine (1 min)" Dim ReadItemArguments4: Set ReadItemArguments4 = CreateObject("OpcLabs.EasyOpc.DataAccess.OperationModel.DAReadItemArguments") ReadItemArguments4.ServerDescriptor.ServerClass = "OPCLabs.KitServer.2" ReadItemArguments4.ItemDescriptor.ItemID = "Simulation.Register_I4" Dim arguments(3) Set arguments(0) = ReadItemArguments1 Set arguments(1) = ReadItemArguments2 Set arguments(2) = ReadItemArguments3 Set arguments(3) = ReadItemArguments4 Dim Client: Set Client = CreateObject("OpcLabs.EasyOpc.DataAccess.EasyDAClient") ' Specify that only synchronous method is allowed. By default, both synchronous and asynchronous methods are allowed, and ' the component picks a suitable method automatically. Disallowing asynchronous method leaves only the synchronous method ' available for selection. Client.InstanceParameters.Mode.AllowAsynchronousMethod = False Dim results: results = Client.ReadMultipleItems(arguments) Dim i: For i = LBound(results) To UBound(results) Dim VtqResult: Set VtqResult = results(i) If VtqResult.Succeeded Then WScript.Echo "results(" & i & ").Vtq.ToString(): " & VtqResult.Vtq.ToString() Else WScript.Echo "results(" & i & ") *** Failure: " & VtqResult.ErrorMessageBrief End If Next
# This example shows how to read 4 items at once synchronously, and display their values, timestamps and qualities. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc # Import .NET namespaces. from OpcLabs.EasyOpc import * from OpcLabs.EasyOpc.DataAccess import * from OpcLabs.EasyOpc.DataAccess.OperationModel import * from OpcLabs.EasyOpc.OperationModel import * # Instantiate the client object. client = EasyDAClient() # Specify that only synchronous method is allowed. By default, both synchronous and asynchronous methods are # allowed, and the component picks a suitable method automatically. Disallowing asynchronous method leaves # only the synchronous method available for selection. client.InstanceParameters.Mode.AllowAsynchronousMethod = False # vtqResultArray = client.ReadMultipleItems([ DAReadItemArguments(ServerDescriptor('OPCLabs.KitServer.2'), DAItemDescriptor('Simulation.Random')), DAReadItemArguments(ServerDescriptor('OPCLabs.KitServer.2'), DAItemDescriptor('Trends.Ramp (1 min)')), DAReadItemArguments(ServerDescriptor('OPCLabs.KitServer.2'), DAItemDescriptor('Trends.Sine (1 min)')), DAReadItemArguments(ServerDescriptor('OPCLabs.KitServer.2'), DAItemDescriptor('Simulation.Register_I4')), ]) for i, vtqResult in enumerate(vtqResultArray): assert vtqResult is not None if vtqResult.Succeeded: print('vtqResultArray[', i, '].Vtq: ', vtqResult.Vtq, sep='') else: print('vtqResultArray[', i, '] *** Failure: ', vtqResult.ErrorMessageBrief, sep='')
System.Object
OpcLabs.BaseLib.Object2
OpcLabs.BaseLib.Info
OpcLabs.BaseLib.Parameters
OpcLabs.EasyOpc.DataAccess.Engine.DAClientMode
OpcLabs.EasyOpc.DataAccess.Engine.EasyDAClientMode